How To Make Material Design Sliding Tabs
In this tutorial we are going to implement sliding tabs in material design style by using the SlidingTabLayout from Google iosched app, The SlidingTabLayout is also provided on the Google developer page as well but it isn't updated with some methods such as setDistributeEvenly() and this method is actually needed to make fixed tabs, the Google ioshed version is more updated and so we are going to use that. Along with the SlidingTabLayout.java we would be needing the SlidingTabStrip.java, Okay so let's start making the Sliding Tabs.
1. Android Studio 1.0.1 (latest)
2. SlidingTabLayout.java & SlidingTabStrip.java from IOsched Google App and copy past both the files in your project
As you can see we have we have made the Linear Layout as the root element for our activity and made the orientation of the LinearLayout as vertical so the elements would be placed one after another, First I have added the toolbar on which I have already made a separate tutorial on how to make a material design toolbar, Next element that I have added is the SlidingTabLayout which acts like a Tab Strip, you can place this strip anywhere in your layout but I want it to be above the pages that are being swiped so I am placing it just below the Toolbar, Next you add the View Pager which is required for swiping the pages. In the code you define every view and then set the SlidingTabs.SetViewPager method to the ViewPager in your activity which is where you tell the app that on page swipe of your View Pager you want the tabs to swipe and change as well.
1. First let's decide the color scheme for our app I want the color scheme to be red so lets do that by making a new color.xml file in your values folder and add the following code to it. You may notice a item in the color.xml with name ScrollColor that is the color which I am going to set to the scroll bar of the Tab Strip.
2. Next we need to make a layout file for toolbar and add that layout file to our apps activity_main.xml. So create a new layout file and name it tool_bar.xml and add the following code to it.
3. Now its time to add Toolbar and the SlidingTabLayout to our main_activity.xml, To add SlidingTablayout in your app you must make sure you have already added the SlidingTabLayout.java and SlidingTabStrip.java in your app as explained in the requirements section at the top. If you have added both the files in your project, open your main_activity.xml and add the following code to it. I have given the SlidigTabLayout an id ="Tabs" and added a elevation of 2dp for the shadow effect.
4. Now we just have to add the ViewPager view to your layout, make sure to give it an Id, I have given my ViewPager an Id called pager. Finally your main_activiy.xml looks something like this.
5. Now I have decided to make 2 tabs for this project, so I have to make the layout for this tabs, so I have created two new layouts tab_1.xml and tab_2.xml and added the medium text view inside both the layout as shown below.
tab_1.xml
tab_2.xml
Now I am done with my layout section and its time to work things out in the code.
6. Now let's first make the 2 fragments for Tab1 and Tab2, So go ahead and create two new java files, name it Tab1 and Tab2 and add the following code to it.
Tab1
Tab2
7. Now before we start working with MainActiviy we need a ViewPager adapter to provide the views for every page i.e every Tab. So go ahead and make a new java file, name it ViewPagerAdapter and add the following code to it. I have added the comments to help you understand the working.
8. Now we have everything ready to start work with the MainActivity.java, So go ahead and add the following code to your MainActivity.java. check out the comments in the code to understand the working.
Now everything is set, Lets just run our app and check out how it looks
Its Looks perfect. Tabs are working nicely. But what if you want to customize the text color of your Tabs Title for a specific event i.e on Page selected etc and you surely would like to tell the user about the active tab he is on. For this you have to make a selector.xml in your res/color/ folder and add the following code to your selector.xml, of course change the colors as your requirements
After making the selector you have to add it in your SlidingTabLayout.java, Look for a method called private void populateTabStrip() and add the following line of code at the end of it.
Look at the SlidingTabLayout code below, I have just copy pasted populateTabStrip() method in which you are suppose to do the changes
Finally, Everything is set and we are done. Let's just run the app and check out how things work.
Well that's just looks amazing, So with this you have learned how to make Sliding Tabs in Material Design style, If you like this tutorial please subscribe to the blog for coming updates.
Requirements
1. Android Studio 1.0.1 (latest)
2. SlidingTabLayout.java & SlidingTabStrip.java from IOsched Google App and copy past both the files in your project
Understanding Sliding Tab Layout Implementation
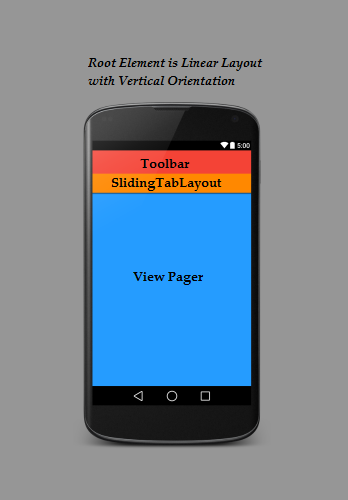
Steps To Implement Sliding Tab Layout
1. First let's decide the color scheme for our app I want the color scheme to be red so lets do that by making a new color.xml file in your values folder and add the following code to it. You may notice a item in the color.xml with name ScrollColor that is the color which I am going to set to the scroll bar of the Tab Strip.
<?xml version="1.0" encoding="utf-8"?> <resources> <color name="ColorPrimary">#e62117</color> <color name="ColorPrimaryDark">#c31c13</color> <color name="tabsScrollColor">#8a140e</color> </resources>
2. Next we need to make a layout file for toolbar and add that layout file to our apps activity_main.xml. So create a new layout file and name it tool_bar.xml and add the following code to it.
<?xml version="1.0" encoding="utf-8"?> <android.support.v7.widget.Toolbar android:layout_height="wrap_content" android:layout_width="match_parent" android:background="@color/ColorPrimary" android:elevation="2dp" android:theme="@style/Base.ThemeOverlay.AppCompat.Dark" xmlns:android="http://schemas.android.com/apk/res/android" />
3. Now its time to add Toolbar and the SlidingTabLayout to our main_activity.xml, To add SlidingTablayout in your app you must make sure you have already added the SlidingTabLayout.java and SlidingTabStrip.java in your app as explained in the requirements section at the top. If you have added both the files in your project, open your main_activity.xml and add the following code to it. I have given the SlidigTabLayout an id ="Tabs" and added a elevation of 2dp for the shadow effect.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <include android:id="@+id/tool_bar" layout="@layout/tool_bar" android:layout_height="wrap_content" android:layout_width="match_parent" /> <com.android4devs.slidingtab.SlidingTabLayout android:id="@+id/tabs" android:layout_width="match_parent" android:layout_height="wrap_content" android:elevation="2dp" android:background="@color/ColorPrimary"/> </LinearLayout>
4. Now we just have to add the ViewPager view to your layout, make sure to give it an Id, I have given my ViewPager an Id called pager. Finally your main_activiy.xml looks something like this.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <include android:id="@+id/tool_bar" layout="@layout/tool_bar" android:layout_height="wrap_content" android:layout_width="match_parent" /> <com.android4devs.slidingtab.SlidingTabLayout android:id="@+id/tabs" android:layout_width="match_parent" android:layout_height="wrap_content" android:elevation="2dp" android:background="@color/ColorPrimary"/> <android.support.v4.view.ViewPager android:id="@+id/pager" android:layout_height="match_parent" android:layout_width="match_parent" android:layout_weight="1" ></android.support.v4.view.ViewPager> </LinearLayout>
5. Now I have decided to make 2 tabs for this project, so I have to make the layout for this tabs, so I have created two new layouts tab_1.xml and tab_2.xml and added the medium text view inside both the layout as shown below.
tab_1.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceMedium" android:text="You Are In Tab 1" android:id="@+id/textView" android:layout_centerVertical="true" android:layout_centerHorizontal="true" /> </RelativeLayout>
tab_2.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceMedium" android:text="You Are In Tab 2" android:id="@+id/textView" android:layout_centerVertical="true" android:layout_centerHorizontal="true" /> </RelativeLayout>
Now I am done with my layout section and its time to work things out in the code.
6. Now let's first make the 2 fragments for Tab1 and Tab2, So go ahead and create two new java files, name it Tab1 and Tab2 and add the following code to it.
Tab1
package com.android4devs.slidingtab; import android.os.Bundle; import android.support.annotation.Nullable; import android.support.v4.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; /** * Created by hp1 on 21-01-2015. */ public class Tab1 extends Fragment { @Override public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { View v =inflater.inflate(R.layout.tab_1,container,false); return v; } }
Tab2
package com.android4devs.slidingtab; import android.os.Bundle; import android.support.annotation.Nullable; import android.support.v4.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; /** * Created by hp1 on 21-01-2015. */ public class Tab2 extends Fragment { @Override public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { View v = inflater.inflate(R.layout.tab_2,container,false); return v; } }
7. Now before we start working with MainActiviy we need a ViewPager adapter to provide the views for every page i.e every Tab. So go ahead and make a new java file, name it ViewPagerAdapter and add the following code to it. I have added the comments to help you understand the working.
package com.android4devs.slidingtab; import android.support.v4.app.Fragment; import android.support.v4.app.FragmentManager; import android.support.v4.app.FragmentStatePagerAdapter; /** * Created by hp1 on 21-01-2015. */ public class ViewPagerAdapter extends FragmentStatePagerAdapter { CharSequence Titles[]; // This will Store the Titles of the Tabs which are Going to be passed when ViewPagerAdapter is created int NumbOfTabs; // Store the number of tabs, this will also be passed when the ViewPagerAdapter is created // Build a Constructor and assign the passed Values to appropriate values in the class public ViewPagerAdapter(FragmentManager fm,CharSequence mTitles[], int mNumbOfTabsumb) { super(fm); this.Titles = mTitles; this.NumbOfTabs = mNumbOfTabsumb; } //This method return the fragment for the every position in the View Pager @Override public Fragment getItem(int position) { if(position == 0) // if the position is 0 we are returning the First tab { Tab1 tab1 = new Tab1(); return tab1; } else // As we are having 2 tabs if the position is now 0 it must be 1 so we are returning second tab { Tab2 tab2 = new Tab2(); return tab2; } } // This method return the titles for the Tabs in the Tab Strip @Override public CharSequence getPageTitle(int position) { return Titles[position]; } // This method return the Number of tabs for the tabs Strip @Override public int getCount() { return NumbOfTabs; } }
8. Now we have everything ready to start work with the MainActivity.java, So go ahead and add the following code to your MainActivity.java. check out the comments in the code to understand the working.
package com.android4devs.slidingtab; import android.support.v4.view.ViewPager; import android.support.v7.app.ActionBarActivity; import android.os.Bundle; import android.support.v7.widget.Toolbar; import android.view.Menu; import android.view.MenuItem; public class MainActivity extends ActionBarActivity { // Declaring Your View and Variables Toolbar toolbar; ViewPager pager; ViewPagerAdapter adapter; SlidingTabLayout tabs; CharSequence Titles[]={"Home","Events"}; int Numboftabs =2; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // Creating The Toolbar and setting it as the Toolbar for the activity toolbar = (Toolbar) findViewById(R.id.tool_bar); setSupportActionBar(toolbar); // Creating The ViewPagerAdapter and Passing Fragment Manager, Titles fot the Tabs and Number Of Tabs. adapter = new ViewPagerAdapter(getSupportFragmentManager(),Titles,Numboftabs); // Assigning ViewPager View and setting the adapter pager = (ViewPager) findViewById(R.id.pager); pager.setAdapter(adapter); // Assiging the Sliding Tab Layout View tabs = (SlidingTabLayout) findViewById(R.id.tabs); tabs.setDistributeEvenly(true); // To make the Tabs Fixed set this true, This makes the tabs Space Evenly in Available width // Setting Custom Color for the Scroll bar indicator of the Tab View tabs.setCustomTabColorizer(new SlidingTabLayout.TabColorizer() { @Override public int getIndicatorColor(int position) { return getResources().getColor(R.color.tabsScrollColor); } }); // Setting the ViewPager For the SlidingTabsLayout tabs.setViewPager(pager); } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.menu_main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); //noinspection SimplifiableIfStatement if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); } }
Now everything is set, Lets just run our app and check out how it looks
Its Looks perfect. Tabs are working nicely. But what if you want to customize the text color of your Tabs Title for a specific event i.e on Page selected etc and you surely would like to tell the user about the active tab he is on. For this you have to make a selector.xml in your res/color/ folder and add the following code to your selector.xml, of course change the colors as your requirements
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:state_selected="true" android:color="@android:color/white" /> <item android:state_focused="true" android:color="@android:color/white" /> <item android:state_pressed="true" android:color="@android:color/white" /> <item android:color="#8a140e" /> </selector>
After making the selector you have to add it in your SlidingTabLayout.java, Look for a method called private void populateTabStrip() and add the following line of code at the end of it.
tabTitleView.setTextColor(getResources().getColorStateList(R.color.selector)); tabTitleView.setTextSize(14);
Look at the SlidingTabLayout code below, I have just copy pasted populateTabStrip() method in which you are suppose to do the changes
private void populateTabStrip() { final PagerAdapter adapter = mViewPager.getAdapter(); final View.OnClickListener tabClickListener = new TabClickListener(); for (int i = 0; i < adapter.getCount(); i++) { View tabView = null; TextView tabTitleView = null; if (mTabViewLayoutId != 0) { // If there is a custom tab view layout id set, try and inflate it tabView = LayoutInflater.from(getContext()).inflate(mTabViewLayoutId, mTabStrip, false); tabTitleView = (TextView) tabView.findViewById(mTabViewTextViewId); } if (tabView == null) { tabView = createDefaultTabView(getContext()); } if (tabTitleView == null && TextView.class.isInstance(tabView)) { tabTitleView = (TextView) tabView; } if (mDistributeEvenly) { LinearLayout.LayoutParams lp = (LinearLayout.LayoutParams) tabView.getLayoutParams(); lp.width = 0; lp.weight = 1; } tabTitleView.setText(adapter.getPageTitle(i)); tabView.setOnClickListener(tabClickListener); String desc = mContentDescriptions.get(i, null); if (desc != null) { tabView.setContentDescription(desc); } mTabStrip.addView(tabView); if (i == mViewPager.getCurrentItem()) { tabView.setSelected(true); } tabTitleView.setTextColor(getResources().getColorStateList(R.color.selector)); tabTitleView.setTextSize(14); } }
Finally, Everything is set and we are done. Let's just run the app and check out how things work.
Well that's just looks amazing, So with this you have learned how to make Sliding Tabs in Material Design style, If you like this tutorial please subscribe to the blog for coming updates.
Thanks man!
ReplyDeleteYour tutorial was very helpfull for me! Very clear and accurate.
Thanks again!
if your project is working fine, please share it with me please
DeleteEmail : Umair_softengr@yahoo.com
Thank you so much! Great tutorial.
ReplyDeleteif your project is working fine, please share it with me please
DeleteEmail : Umair_softengr@yahoo.com
Thanks a lot !
ReplyDeleteGreat tutorial, very helpful and brightly made !
if your project is working fine, please share it with me please
DeleteEmail : Umair_softengr@yahoo.com
Awesome tutorial, thanks! :-) .... Could be next lesson open new activity (this 2-tab activity) from left drawer from previous tutorial? ;-)
ReplyDeleteGreat tutorial. Thanks. Taking in account that Pre-Lollipop doesn't allow properties like 'android:elevation="2dp"', what is the best way to simulate it for API lower than 21?
ReplyDeleteThanks.
i guess you can use shadows, which can be abit tricky :D
DeleteYou made very usefull tutorial! Thanks!
ReplyDeleteif your project is working fine, please share it with me please
DeleteEmail : Umair_softengr@yahoo.com
thanks gud tutorial
ReplyDeleteif your project is working fine, please share it with me please
DeleteEmail : Umair_softengr@yahoo.com
This was exactly what I was looking for, Thanks! I seem to be having some errors tho, is there anyway I could get the source code? :D
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteWell explained!
ReplyDeleteFor some reason my app would not work and always crashes moment I launch it. I followed the tutorial and restarted the app thee times from scratch and still would not work. Is there something I should change that you haven't mentioned in the tutorial? Please let me know. thanks.
ReplyDeleteIf you are using AppCompat, in styles.xml put this line
Deletefalse
Hey ! Facing the same problem. Did you find any solution?
Deletein main_activity.xml change com.android4devs.slidingtab to your package name
DeleteThis comment has been removed by the author.
DeleteChanges to be made:
DeleteIn main_activity.xml
Change com.android4devs.slidingtab to your package name.
In style.xml
item "windowActionBar" as false
item "windowNoTitle" as true
My app created jst lyk above procedure doesnt opens, force stops while opening itself. Any idea sir?
ReplyDeletein main_activity.xml change com.android4devs.slidingtab to your package name
Delete@mehviish I tried the change, my app still crashes. all code is same to same, please help
DeleteThis comment has been removed by the author.
ReplyDeleteHi Akash,
ReplyDeleteThanks for the tutorial. Is there anyway I can add badge to tabs to show users count?
Awesome tutorial! Thanks man! Happy coding!! ;D
ReplyDeleteI got an Error " HorizontalScrollView can host only one direct child"
ReplyDeleteHi
ReplyDeletePlease tell me if it's work for API 16 (android 4.1.2) thank you
Thanks a lot, especially for the part about coloring text in tabs!
ReplyDeleteHey I loved your tutorial and thanks for your effort. I just have one error in the ViewPagerAdapter Class of the public fragment getItem method. the error is about returning the tab1 and i don't know how to get my head round it. the error states "Incompatible type: Required: android.support.v4.app.Fragment
ReplyDeleteFound: com.slidingtoolbar.Tab1"
Tab1 needs to extend android.support.v4.app.Fragment class
DeleteThis comment has been removed by the author.
ReplyDeleteThanks and love your tutorial.... Finally I able to create this design based on your tutorial.... Thank you very much... Would like to see more of your tutorial!!!
ReplyDeleteWondering is it possible for you to provide some tutorial for the animations?
ReplyDeleteHi Akash,
ReplyDeleteThank you for the tutorial!
I have an issue, I need to use the OnResume(); method in my fragments, but when I switch between the tabs, the method isn't called, could you help me please?
Thanks!
I found the answer:
Deletehttp://stackoverflow.com/questions/10024739/how-to-determine-when-fragment-becomes-visible-in-viewpager
This comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteHi Akash. Thanks for great tutorial.
ReplyDeleteI just have an improvement. Instead of modifying SlidingTabLayout.java to set text color and size you can use setCustomTabView method.
mTabLayout.setCustomTabView(R.layout.tab_indicator, android.R.id.text1);
tab_indicator layout:
TextView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@android:id/text1"
android:background="?android:selectableItemBackground"
android:layout_width="wrap_content"
android:paddingLeft="16dp"
android:paddingRight="16dp"
android:layout_height="48dp"
android:gravity="center"
android:textColor="@color/selector"
android:textSize="14sp"
android:textStyle="bold"
android:fontFamily="@string/font_fontFamily_medium"
android:textAllCaps="true"
This comment has been removed by the author.
ReplyDeleteI have copy pasted the entire code in my Android studio. No errors during build.
ReplyDeleteWhenever I run the app, it crashes even before it opens.
Please help.
Change the package name in activity_main.xml to your package name.
DeleteIn style.xml add
item name="windowActionBar" as false
item name="windowNoTitle"as true
You have to make color directory. Inside that you have to paste the selector file.
Tabs are not visible in my app but sliding functionality is working.
ReplyDeleteplease do help
How can I decrease the tab size?
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThank you so much. Very neat explanation & your tutorial has helped me a lot in making tabs :-) God bless u :-D Keep coding :-) Arigato Gozaimasu.
ReplyDeleteThis Tutorial Was damn Good .I need the same integrated in NAvigation drawer.Can you please
ReplyDeleteI used these tabs with a navigation drawer. I can send you the code if you want..
DeleteCan you please send it to me?
DeleteThis comment has been removed by the author.
DeleteI also want to integrate this code in Navigation Drawer.. do you know how?
DeleteI also want to integrate the sliding tab thing in Navigation drawer. Please can you send me the code?
DeleteHello.
ReplyDeleteI have 3 tabs and each goes into a separate detail activity.
However if I press the back button it always goes to the first tab, instead of the tab that started the activity.
Is there a way to go to a certain tab programatically? I'm thinking of storing the tab # , and after pressing the back button calling a goToTab(number) (for example) to return to that tab and not the starting activity which contains the tabs.
Can i get the answer for this? I also want to do this.
DeleteThis comment has been removed by the author.
ReplyDeleteI am novice into android..
ReplyDeleteI am getting eroor at : android:theme="@style/Base.ThemeOverlay.AppCompat.Dark"
-- No resource found that matches the given name
how should i do this step?
ReplyDelete2. SlidingTabLayout.java & SlidingTabStrip.java from IOsched Google App and copy past both the files in your project
Should i create a class and copy the codes ?
yes!Create this classes and just copy&paste them
DeleteThis comment has been removed by the author.
ReplyDeleteHi. i have a problem when i try create 5 tabs with table layouts inside them. First, the 3rd table returns null on findviewbyid. And second when i implement only the first two tabs and click on the 3rd one the first tab looses its contents. Any idea about this?
ReplyDeletefix this. i should set setOffscreenPageLimit(5) for 5 tabs...:)
DeleteHi men i have an error when launch the app. "This Activity already has an action bar supplied by the window decor. Do not request Window.FEATURE_ACTION_BAR and set windowActionBar to false in your theme to use a Toolbar instead."
ReplyDeleteThe answer is on his previous Toolbar tutorial and I quote:
Delete"3. Even though ActionBars are replaced by App bar/Toolbar we can still use ActionBar, but in our case we are going to make a Toolbar so go to your style.xml and change the theme to "Theme.AppCompat.Light.NoActionBar, This helps us get rid of the ActionBar so we can make a App bar."
This comment has been removed by the author.
ReplyDeleteHi
ReplyDeletecan you help me?
I want display tab in fragment.
i do but it not display.
this is HomeFragment
http://i.imgur.com/ckcgDTU.jpg
This comment has been removed by the author.
ReplyDeleteHi
ReplyDeleteFirst Thank you men!!!
Second How to change the background color of SlidingTabLayout by state "pressed, selected, unselected"? I'm try by hours but i can't make it works.
This comment has been removed by the author.
Deleteif you want change text color of background tab, you can do this changing value of <item android:color="here" /> in res/color/selector.xml
DeleteThanks for the good tutorial. You are the best :)
ReplyDeletehey thank you for the awesome tutorial!! i did exactly as u told!! but wen i run it on ma phone, a message prompts saying app has unfortunately stopped ! if anyone has the app working perfectly!! plz do mail it to me!
ReplyDeleteat lijodavidson@gmail.com
thanks
Thanks for this good tutorial!! Great one!
ReplyDeletehi, I followed this tutorial, everything works fine but when I use a listview into a slidingtab the text (in the listview) turns white whereas it was black... do you have an explanation
ReplyDeleteHi, thanks for such a great piece of code. I implemented this in one of my project. However I am having an issue.
ReplyDeleteI am showing three tabs. In one tab I am calling api and populating the data in listview. In second tab I am getting data from db and populating it in listview. In third tab I am showing list of contacts from phonebook. Now because these heavy computations on my main thread, the ViewPager is not scrolling fast and smoothly. Its lagging.
How can I avoid it? When and how should I load and display data to make my ViewPager scroll like lightening?
Hi, I also want to do something similar, can you help?
DeleteDear Author,
Can you produce a tutorial for SlideTabs with ListView?
Good Tutorial!!
ReplyDeleteI am having an issue here. "R.color.selector" is not working. It is added in drawable folder and can't be added in values folder.
Oh! I have done it. You need to create "color" sub directory under "res" directory and then add "selector.xml" there. Thanks
DeleteAwesome tutorial. Congrats!
ReplyDeleteI need to use these tabs with a navigation drawer. Do you have anything related to help me?
Thanks!
Does this example works on pre lollipop devices. For lollipop devices it's working fine but on kitkat it's not
ReplyDeleteHello,
ReplyDeleteWhen I go landcape : it not work. I crreate a layout for a view specially to landscape mode and not use it ? Why ?
Hello people great guide. Thanks
ReplyDeleteI have done everything as described but app crashes on every device where i tested.
If someone could send me working code, that would be awesome! Thanks
Oh mail is mile.vrtunic@gmail.com
Excuse me, I tried the codes above, but I got some error.
ReplyDeleteCould you upload your whole project on the web ,please?
Let's us download it!
Thanks a lot.
hey,
ReplyDeletei want to make the textview of the selected tab bold like you did with the textColor, but i cant use a selector for that. do you know a way to that. making the selected tab textStyle Bold.
Hi Akash. Thank you for your great website.
ReplyDeleteI would be honored if you can help me , how can I set swipe tabs slide right to left ?
Hey dude,
ReplyDeleteI've got your example code up and running within my current app, it works great the first time around, but when navigating to another fragment and then back again the tab fragments are empty.
Any ideas what might be causing this?
What should i write in Fragment getItem method if i want to add one more tab?
ReplyDeleteThank you for this tutorial.
In your Fragment getItem change it to this:
Delete@Override
public Fragment getItem(int i) {
switch (i) {
case 0:
return new Tab1();
case 1:
return new Tab2();
case 2:
return new Tab3();
case 3:
return new Tab4();
}
return null;
Make sure you add new Java,
In your MainActivity change CharSequence and int Numboftabs to(or what ever you want):
CharSequence Titles[]={"Home","Events","Test 1","Test 2"};
int Numboftabs =4;
Thanks dude for the tutorial, but i'm facing little problem. The sliding tabs is too large in height (since use property wrap content) and its title for each tabs did not show.
ReplyDeleteI have read your tutorial many times to see what I missed, but I couldn't find it.
Can ou give me solution?
Thank you very much.
you can use improvements of "Roland Yeghiazaryan" http://www.android4devs.com/2015/01/how-to-make-material-design-sliding-tabs.html?showComment=1426238480095#c6242495915977088200
DeleteI just have an improvement. Instead of modifying SlidingTabLayout.java to set text color and size you can use setCustomTabView method.
mTabLayout.setCustomTabView(R.layout.tab_indicator, android.R.id.text1);
tab_indicator layout:
<TextView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@android:id/text1"
android:background="?android:selectableItemBackground"
android:layout_width="wrap_content"
android:paddingLeft="16dp"
android:paddingRight="16dp"
android:layout_height="48dp"
android:gravity="center"
android:textColor="@color/selector"
android:textSize="14sp"
android:textStyle="bold"
android:fontFamily="@string/font_fontFamily_medium"
android:textAllCaps="true"/>
change layout_height to "wrap_content" and add android:paddingBottom="you chose value"
how to use this tab view in a fragment....
ReplyDeleteI want to use it with navigation drawer and i use a modules for navigation drawer ...
please help me anyone........
hi!
ReplyDeletei successfuly do all this stuff but i gor a problemm, here is description:
http://stackoverflow.com/questions/30647339/missing-slidingtablayout?noredirect=1#comment49393384_30647339
will be glad any solutions!
Hello, how to se Tag for the each fragment?
ReplyDeletehello, how to set tag for each fragment and get current visible fragment?
ReplyDeletehello, have a source code ?
ReplyDeleteHi, Fabulous tutorial. I have followed it step by step, and made it to the point where my code seems to have no errors, it compiles sweet. But as soon as I run it on emulator it crashes with the following message : "Unfortunately, Testing_sidebar has stopped" (Testing_sidebar is the name of the project) . COuld you help me with what would be the error ?
ReplyDeleteIn your activity_main.xml, try to rename <com.android4devs.slidingtab.SlidingTabLayout... with your own package name like this : <com.yourpackage.SlidingTabLayout...
DeleteQAQ Thanks a lot.
DeleteThank you so so much for this tutorial. It helped me out a lot. Works perfectly.
ReplyDeleteHi faboulous tutorial nut i am facing an error while running the project. The error is below.
ReplyDeletejava.lang.NullPointerException: Attempt to invoke virtual method 'void android.support.v4.view.ViewPager.setAdapter(android.support.v4.view.PagerAdapter)' on a null object reference
This comment has been removed by the author.
DeleteI got the same problem, maybe you can check your layout, I solve it like this
Delete@Overrid
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout."YOUR_LAYOUT_NAME");
...
}
great tutorial. how could I add images instead of text on the tab.??
ReplyDeleteGreat tutorial..
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteHi!
ReplyDeleteGetting this error:
"Unable to start activity ComponentInfo{name_of_app/name_of_app.MainActivity}: android.view.InflateException: Binary XML file line #68: Error inflating class fragment"
This comment has been removed by the author.
Deletein activity_main.xml
Deletereplace `com.android4devs.slidingtab` with your package name
I have a button on first tab and on click of that button I have to navigate to Tab2. How can I do that?
ReplyDeleteDude cannot start activity from your fragment.....Guide us through...........
ReplyDeleteDude cannot start activity from your fragment.....Guide us through...........
ReplyDeleteDude cannot start activity from your fragment.....Guide us through...........
ReplyDeleteHI!! I want to draw a simple partition line between tabs. How I would be able to achieve this. Please help me.
ReplyDeleteHi i have encountered error Unable to start activity ComponentInfo{com.cap2.macsanity.droidgency/com.cap2.macsanity.droidgency.MainActivity}: android.view.InflateException: Binary XML file line #2: Error inflating class how to fix this? please help me
ReplyDeleteWhy do have to use external library to have swipe effect? Is there any way to use tablayout directly with all functionalities by using the support library itself?
ReplyDeleteI using sliding tabs in setting screen of live wallpaper using Fragment Activity and it have tabs but swiping doesn't work perfectly. I have total 3 tabs and have to swipe 6-7 times to reach to 3rd tab through swipe. can you please help?? Min api version is 9 and max api is 22
ReplyDeletesaya mendapatkan error pada saat run
ReplyDeletewarning : the project encoding (windows-1252) does not match the encoding specified in the gradle build files (UTF-8)
This Tutorial was good. Can we integrate navigation drawer to this ?
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteShow de bola. Perfeito!!!
ReplyDeletePlease Help...
ReplyDeleteI got Rendering error when i try to create tool_bar.xml
"Rendering Problems The following classes could not be found:
- android.support.v7.widget.Toolbar (Fix Build Path, Create Class)
Tip: Try to build the project. "
How to solve this error I tried alot.
Dont tell me to move on lower API
Hi, thanks for this tutorial, with the help of this am learning the concept of SlidingTabs using view pager, once am implemented am get the issues on following lines
ReplyDeleteif (mDistributeEvenly) {
LinearLayout.LayoutParams lp = (LinearLayout.LayoutParams) tabView.getLayoutParams();
// lp.width = 0;
// lp.weight = 1;
}
Once am comment the width and weight means am able to run it and the tabs are displayed nearby each other not as like as it fit for the screen because of the weight am comment it.Will you please guide me how to rectify this.
if you want both centered just set mDistributeEvenly to true (OR comment out the "if(mDis...)" lp.weight needs to be 1 that both textviews will use the space they can fill.
Delete----TAB 1---- | ----TAB 2---- // <- result when weight is set
TAB1 | TAB2 // <- result if NOT
Hope that make sense? :)
Can you share a working project to abhimaliyeckal@gmail.com plz ?
ReplyDeleteany help appreciated :)
Hi, i've my shared my code via mail, thanks
ReplyDeleteHow to Add sliding tab in Naviagation
ReplyDeletehi, is it able to add images in the place of tabs heading section
ReplyDeleteHi there i put listview in tab1 which is Newsfeed in my code. the problem is its showing the Newsfeed not my tab2 which is Inbox here is my codes pls help me
ReplyDelete@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View v = inflater.inflate(R.layout.activity_newsfeed, container, false);
listView = (ListView) getActivity().findViewById(R.id.list);
feedItems = new ArrayList<>();
listAdapter = new FeedListAdapter(getActivity(), feedItems);
listView.setAdapter(listAdapter);
// new ColorDrawable(getResources().getColor(android.R.color.transparent)));
// We first check for cached request
Cache cache = AppController.getInstance().getRequestQueue().getCache();
Cache.Entry entry = cache.get(URL_FEED);
if (entry != null) {
// fetch the data from cache
try {
String data = new String(entry.data, "UTF-8");
try {
parseJsonFeed(new JSONObject(data));
} catch (JSONException e) {
e.printStackTrace();
}
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
} else {
// making fresh volley request and getting json
JsonObjectRequest jsonReq = new JsonObjectRequest(Request.Method.GET,
URL_FEED, null, new Response.Listener() {
@Override
public void onResponse(JSONObject response) {
VolleyLog.d(TAG, "Response: " + response.toString());
if (response != null) {
parseJsonFeed(response);
}
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
VolleyLog.d(TAG, "Error: " + error.getMessage());
}
});
// Adding request to volley request queue
AppController.getInstance().addToRequestQueue(jsonReq);
v.bringToFront();
}return v;
}
/**
* Parsing json reponse and passing the data to feed view list adapter
* */
private void parseJsonFeed(JSONObject response) {
try {
JSONArray feedArray = response.getJSONArray("feed");
for (int i = 0; i < feedArray.length(); i++) {
JSONObject feedObj = (JSONObject) feedArray.get(i);
FeedItem item = new FeedItem();
item.setId(feedObj.getInt("id"));
item.setName(feedObj.getString("name"));
// Image might be null sometimes
String image = feedObj.isNull("image") ? null : feedObj
.getString("image");
item.setImge(image);
item.setStatus(feedObj.getString("status"));
item.setProfilePic(feedObj.getString("profilePic"));
item.setTimeStamp(feedObj.getString("timeStamp"));
// url might be null sometimes
String feedUrl = !feedObj.isNull("url") ? feedObj
.getString("url") : null;
item.setUrl(feedUrl);
feedItems.add(item);
}
// notify data changes to list adapater
listAdapter.notifyDataSetChanged();
} catch (JSONException e) {
e.printStackTrace();
}
where to set mTabViewLayoutId? I want to have a layout at tab
ReplyDeletereally nice tutorial , please tell me how to change the height of sliding tab strip
ReplyDeleteGreat tutorial. Thank you!
ReplyDeletehow can i use customtabs with two textviews in vertical .... i have provieded id of layout but still it showing me one textview in tabs
ReplyDeleteHello all,
ReplyDeletethis tutorial works well on lollipop devices, but on pre-lollipop devices i cant move to next tab after click on tab name, only way how to move to next tab is swipe. So please can anyone tell me how to make tab names clickable on pre-lollipop? Thanks in advance.
Honza
This comment has been removed by the author.
ReplyDeleteSpend a whole day , and not working at all!
ReplyDeleteAwesome tutorial , thanks a lot !
ReplyDeleteBut there are some problems with the "Subscribe System", I couldn't receive any Confirm E-mail after subscribed.(foxmail)
Hello your project with this article it working if working please send me the project simple. thank u
DeleteThanks Dude !!!
ReplyDeleteReally Helpful, You Saved me. I was in trouble from two days.
Hello!! if your project is working fine, please share it with me please
DeleteEmail :vongchamnanz@gmail.com
Hey guys. Is there a way of knowing what tab we are on from Main activity? Because I want some FAB animations.
ReplyDeleteThanks, very clear, very detailed.
ReplyDeleteHello your project with this article it working if working please send me the project simple. thank u
DeleteWow!! Now I can do it complete Thank q so much.
ReplyDeleteSo I have one quest to ask :
how to add Icon to each Tab??
Please feedback to me : vongchamnanz@gmail.com
Hey i know this is a bit late but I'm getting an error. So when i try to have more than two tabs like for say a 3rd tab if i swipe to anything other than the first tab , the tab will load then after its finish loading it will crash with a .NullPointerException I can't seem to figure out what i may have missed.
ReplyDeleteDear Author,
ReplyDeleteCan you produce a tutorial for Swipe tabs with ListView?
How to set Icons instead of tab name??
ReplyDeleteVery nice tutorial
ReplyDeleteVery nice and helpful tutorial. But I have a problem using this. It seems that If lets say we have more than 2 tabs, and we want to refresh them, when you go from A to B and back to A it is not refreshed cause the ViewPager uses pipeline. Do you have any suggestions?
ReplyDeletegetting error the activity already has an action bar supplied by window decor do not request window.Feature_Action_Bar and set Window action bar as false
ReplyDeleteHello i included my package name as well as i included the item name in my styles.xml file but my app crashes on launch. Please provide me solution asap
ReplyDeleteOnly creates an instance two fragments at a time , then press the other when going moving , how to increase ?
ReplyDeleteOnly creates an instance two fragments at a time , then press the other when going moving , how to increase ?
ReplyDeleteInstead of titles, I wanna show icon images as titles like in facebook in the SlidingTabLayout title, how to do that??
ReplyDeleteHi
ReplyDeleteVery great tut , very clear explannation.
I want to know if it will work for android min SDK = 10?
Now can you help me to make a click event for a button which is inside tab_1.xml or tab_2.xml ?
ReplyDeleteAwesome tutorial it was.
It's a Wonderful tutor...
ReplyDeleteI have four Tabs like A,B,C and D
a ViewPager loads the next view so that it is ready when you want to scroll to the next fragment. by default the viewpager loads the previous current and next fragment in the ViewPager so when you scroll from A to B, C is going to be loaded in so that you have a smooth scroll when you go to C
But My C Fragment's input is come after update the B Fragment So, C Frgament's OnCreate Doesn't work again.. Do you have any idea to do that? Please share with me...
Thanks
Tabs UI Design: Click Here
ReplyDeletethanks dear but asked you one thing more how to change tabs name or text with icons on the tabs??
ReplyDeletehi...
ReplyDeleteNice tutorials...
Bt How to change sliding tab text color when its click?i am using populateTabStrip() in my code but its not work.
Thank you
Great Tutorial !!!
ReplyDeleteBest Android Tabs Libraries: Click Here
ReplyDeleteThank`s man
ReplyDeleteHi
ReplyDeletei have a couple of problems,
The first one is about the menu_main. I didnt have that so i created one in the menu folder, but empty must i put something in there?
The other problem is about the R.id.action_settings, there is no id with that name, so i created in a folder called ids
ofc it didnt worked. What i have to do¿
My app just say that unfortunately the app ahs stopped.
Do you need more info to tell me what is wrong? just tell me.
Thank you so much in advance!
Regards
okey
Deletei put something like this:
inside menu_main.xml and the problem disappear but it still is saying that unfortunately the app has stopped idk where is the problem :(
Just remove the lines showing the errors. You don't need them.
Deletewhat do you mean?
Deleteremove this:
override
public bolean...
(R.menu_menumain..)
...
and
public bloean
int id = item.getitemid..
if (id == action.settings)...
...
Bro,
ReplyDeleteYou really rock! I removed some things such as the toolbar, as it was taking some extra space and honestly the tutorial is really good!
Thanks a lot!
Rendering Problems The following classes could not be found:
ReplyDelete- com.android4devs.slidingtab.SlidingTabLayout (Fix Build Path, Create Class)
Tip: Try to build the project.
Please help me
In activity_main.xml replace "com.android4devs.slidingtab.SlidingTabLayout" with the path to your class.
DeleteThanks. You are awesome
ReplyDeleteThis comment has been removed by the author.
ReplyDeletecan u please send me zip file to me this example pavansiva999@gmail.com
ReplyDeleteThank You!! This was awesome (y)
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeletehi, how can i do this in only one half of the screen,,like the bottom side,,and the top i will have other stuff like images that the user can change when he presses the right or left button, and the slide tabs should be on the other half....
ReplyDeleteHey, everything works perfect but where the colorprimarydark is implemented? My upper side of page still looks gray(where the phone icons stay) Also, getcolorstatelist(int id) is deprecated do you have any alternative for that? Thank you so much.
ReplyDeletehow can i import SlidingTabLayout in android studio
ReplyDeletehello,
ReplyDeletei wanna start tabs from right for arabic language(right align) (in ordinary situation there start from left)
any body can help me please :)
Thank you! Helps me a lot!
ReplyDeleteAndroid Tutorial: viralandroid.com/2015/11/android-tutorial.html
ReplyDeleteThanks! But how can i change the color of tabs strip?
ReplyDeleteHi,if your project is working fine, please share it with me please Email :jonewonewtest@gmail.com Thank You.
ReplyDeleteThank you so much! Muito obrigado!
ReplyDeleteGood Tutorial...
ReplyDeleteTHXS VERY GOOD TUTORIAL :)
ReplyDeleteVery helpful.
ReplyDeleteMade my work so much faster.
thanks.......waiting for more tutorial
ReplyDeletecan I have anyone working project. I would really appreciate that
ReplyDeletekhairulizha95@gmail.com
Can u please update its manifest.xml file, coz getting an errors of unfortunate application stopped.
ReplyDeleteHi, why i have this problem on MainActivity?
ReplyDeletehttp://i.imgur.com/D6II83p.png
Hi ,
ReplyDeleteI have used your code to show different fragment in home page with one addition of navigation drawer.
I want to open different fragment/ view in navigation drawer on home page.
Please suggest me the way to open different view in the navigation drawer based on view pager position.
Hey! Gr8 tutorial. It's working very fine for me.
ReplyDeleteSo now I want to add recyclerView under the tabs. Can you or anybody can help me with this? I want to know how to implement recyclerview inside fragments.
Thanks
how to add icon to these tabs
ReplyDeleteI'm having trouble with only one part but I can't seem to fix/solve it, its on the MainActivity with the getMenuInflater().inflate(R.menu.menu_main, menu); and R.id.action_settings (i.e last two methods of MainActivity). I don't have a res/menu file.
ReplyDeleteDoes anyone how to fix this or info on what to place in the menu.xml file?
I'm having trouble with only one part but I can't seem to fix/solve it, its on the MainActivity with the getMenuInflater().inflate(R.menu.menu_main, menu); and R.id.action_settings (i.e last two methods of MainActivity). I don't have a res/menu file.
ReplyDeleteDoes anyone how to fix this or info on what to place in the menu.xml file?
hey, I have a problem in ViewPagerAdapter. error in "return tab1" and "return tab2" which is position value. It shown as incompatible types.
ReplyDelete