How To Make Material Design App Bar/ActionBar and Style It
In this article and most of the coming articles we would get started with material design, Material design brings lot of new features to android and In this article we would see how to implement App Bar which is a special type of ToolBar (ActionBars are now called as App Bars) and how to add actions icons to App Bar
Before we start make sure you have these requirements fulfilled.
1. Android Studio 1.0.1 (Latest while writing the post)
2. Appcombat v7 Support library (To Support Pre Lollipop devices)
If you have the Android Studio 1.0.1 then you don't need to worry about Appcombat v7 support library as it comes with the compiled dependency of latest Appcombat support library. Before we start coding let's look at what are we trying to make.
Lets Start,
1. Open Android Studio and create a new project and select a blank activity to start with.
2. If you are on the latest version of Android Studio you don't have to add a compiled dependency of Appcombat v7 21 if not then please make sure you add the line below in your gradel build dependencies.
3. Even though ActionBars are replaced by App bar/Toolbar we can still use ActionBar, but in our case we are going to make a Toolbar so go to your style.xml and change the theme to "Theme.AppCompat.Light.NoActionBar, This helps us get rid of the ActionBar so we can make a App bar.
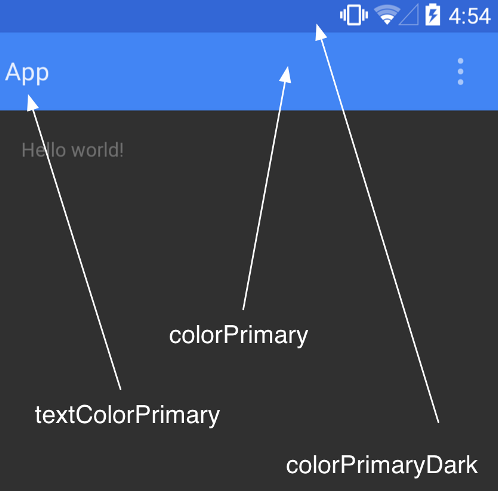
To do that you need make a file called color.xml in your values folder and add the color attributes as shown in the below code.
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="ColorPrimary">#FF5722</color>
<color name="ColorPrimaryDark">#E64A19</color>
</resources>
And this is how the style.xml looks after adding the colors.
<resources>
<!-- Base application theme. -->
<style name="AppTheme" parent="Theme.AppCompat.Light.NoActionBar">
<item name="colorPrimary">@color/ColorPrimary</item>
<item name="colorPrimaryDark">@color/ColorPrimaryDark</item>
<!-- Customize your theme here. -->
</style>
</resources>
5. Now lets make a Toolbar, Toolbar is just like any other layout which can be placed at any place in your UI. Now as the toolbar is going to be needed on every or most of the activities instead of making it in the activity_main.xml we would make a separate file called tool_bar.xml and include it in our activity this way we can include the same file on any activity we want our toolbar to appear.Go to res folder in your project and create a new Layout Resource File and name it tool_bar.xml with the parent layout as android.support.v7.widget.Toolbar as shown in the image below.
6. now add the background color to your tool_bar as the primary color of the app and give an elevation of 4dp for the shadow effect, this is how the tool_bar.xml looks.
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.Toolbar xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/ColorPrimary"
android:elevation="4dp"
>
</android.support.v7.widget.Toolbar>
7. Now let's include the toolbar we just made in our main_activity file, this is how the main_activiy.xml looks.<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<include
android:id="@+id/tool_bar"
layout="@layout/tool_bar"
></include>
<TextView
android:layout_below="@+id/tool_bar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="@dimen/TextDimTop"
android:text="@string/hello_world" />
</RelativeLayout>
At this point this is how the app looks like as below.
8. As you can see, the tool_bar layout is added, but it doesn't quite look like a Action Bar yet, that's because we have to declare the toolBar as the ActionBar in the code, to do that add the following code to the MainActivity.java, I have put comments to help you understand what's going on.
package com.example.hp1.materialtoolbar;
import android.support.v4.widget.DrawerLayout;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.support.v7.app.ActionBarDrawerToggle;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.support.v7.widget.Toolbar;
import android.view.Menu;
import android.view.MenuItem;
import android.view.MotionEvent;
import android.view.View;
import android.widget.Toast;
public class MainActivity extends ActionBarActivity { /* When using Appcombat support library
you need to extend Main Activity to
ActionBarActivity.
*/
private Toolbar toolbar; // Declaring the Toolbar Object
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
toolbar = (Toolbar) findViewById(R.id.tool_bar); // Attaching the layout to the toolbar object
setSupportActionBar(toolbar); // Setting toolbar as the ActionBar with setSupportActionBar() call
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
After that, this is how the ToolBar looks
9. Notice that the name of the app "MaterialToolbar" is black as we have set the theme parent to Theme.AppCompat.Light.NoActionBar in step 3 it gives the dark text color, So if you want to set the name as light/white text then you can just add android:theme="@style/ThemeOverlay.AppCompat.Dark" in tool_bar.xml and you would get a light text color for the toolbar text, So finally tool_bar.xml looks like this.
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.Toolbar xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/ColorPrimary"
android:theme="@style/ThemeOverlay.AppCompat.Dark"
android:elevation="4dp"
>
</android.support.v7.widget.Toolbar>
10. All left is to show you how to add menu items like search icon and user icon, for the icons i use icons4android.com which is free to use and I have also mentioned it in my 5 tools every android developer must know Post. I have downloaded the icons in four sizes as recommended by the official Google design guideline and added them to the drawables folder. You can see the project structure from image below.
11. now I need to add search icon and user icons in the menu_main.xml as shown below
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
tools:context=".MainActivity">
<item
android:id="@+id/action_settings"
android:orderInCategory="100"
android:title="@string/action_settings"
app:showAsAction="never" />
<item
android:id="@+id/action_search"
android:orderInCategory="200"
android:title="Search"
android:icon="@drawable/ic_search"
app:showAsAction="ifRoom"
></item>
<item
android:id="@+id/action_user"
android:orderInCategory="300"
android:title="User"
android:icon="@drawable/ic_user"
app:showAsAction="ifRoom"></item>
</menu>
And now everything is done our App bar looks just how we wanted it to look.
So finally we have done and our App Bar looks just how we had seen above and as we have used the appcombat support library it is compatible on pre lollipop devices as well, hope so you liked the post, if you did please share and comment.
Nice Article
ReplyDeleteThank you, stay tuned as lot more material design tutorials and tips are coming soon.
DeleteVery good tutorial! Thanks!
DeleteGreat tutorial. One element I couldn't find was from your activity_main.xml file where you cite:
ReplyDeleteandroid:layout_marginTop="@dimen/TextDimTop"
What is the correct value to place in the dimens.xml file? I assumed it was small so I just guessed 12dp. Other than that, thanks for your hard work as this was one of the most clearly written tutorials I have come across.
Hey Kevin,
DeleteI am really glad that you liked the tutorial, The value for TextDimTop that I have used is 5dp.
Awesome, thanks for the quick reply! You write some of the most clearly written tutorials out there so keep up the good work.
DeleteHey Kevin,
DeleteThank you for your comment and I would surely continue to update the blog with latest and interesting tutorials, In the mean time if you want any specific tutorial feel free to let me know, you can get the contact information on the contact us page. Till then happy coding.
Nice article! Plan on using this and your Material Navigation Drawer in my first app!
ReplyDeleteOh, and I had a question. I noticed when changing the text color to white on the toolbar, the text only becomes white in Lollipop, not Kitkat. Any way to make it white in Kitkat as well?
DeleteYou have make a different style.xml for kitkat version and set the theme for toolbar as your requirement
DeleteThanks so much for this! I haven't been able to find many material design tutorials yet so this is really helpful. I was wondering if there was any way to get 'elevation' working on pre-lollipop devices. Without being able to layer elements, material design isn't much. Thanks again!
ReplyDeleteWelcome, Please make sure you subscribe to the blog for upcoming tutorials on material design, and regarding your question, yes you can probably get a elevation type of effect in kitkat by making a custom drawable with the shadow effect and making that drawable the background of the view you want the elevation to be applied to.
DeleteWhenever I add setSupportActionBar(toolbar); in MainActivity.java as shown above , error comes " setSupportActionBar (android. support. v7.widget.Toolbar) in ActionBarActivity cannot be applied to (android. widget.Toolbar) ' please help , I am using android studio 1.0.2
ReplyDeleteHi, I was having the same problem, solution here:
Deletehttp://stackoverflow.com/questions/29025961/setsupportactionbar-toolbar-error
Hi!
ReplyDeleteThanks for your tutorial!
I'm trying to change the menu background color to a custom one, but I'm finding it impossible... I've been trying many methods, but nothing happens. I'm about to surrender. Any idea on how to change the menu's background color?
Thanks
Great article, easy to follow.
ReplyDeleteHowever the drawer is not overlapping my action bar (that is my goal) .... my min is SDK 14, any thoughts ?
This comment has been removed by the author.
ReplyDeleteWhat should I do if i got an error saying "element span must be declared"?
ReplyDeleteHi, I had the same doubt, just dont use it.. if you're copy-pasting the code, just omits < span> element..
DeleteThanks for this, very helpful! Just one thing I found when following this guide: using the android:theme attribute to set the toolbar text colour only works on Lollipop devices. Using app:theme works on both Lollipop and pre-Lollipop. I also used app:popupTheme and it works just as well.
ReplyDeleteThx ! It's a great article !
ReplyDeleteBut when I use "span", Android Studio tell me : "Element span must be declared" ! Why ?
span tag belongs to HTML.. Just delete it...
Deletewhy dont you put source code? :D
ReplyDeleteThank you so mach!
ReplyDeleteHow can I provide support for older versions of Android? I'm talking about android:elevation=" ". When I use it, I don't see any shadows on my phone (android 4.2)
ReplyDeleteSame problem
DeleteHow to use the android:actionLayout menu item attribute to provide a custom view layout for the action item?
ReplyDeleteHi, Excellent Tutorial ! I have a question, I've done everything you have here and it works, except that I can't see the "ColorPrimaryDark" at the top.. it's the same "black" as always :( please help.
ReplyDeleteThanx!
The same problem!
DeletePlz, reply if you find solution
DeleteI have the same problem. What would be the reason?
DeleteI have same problem .. plzz any one reply
DeleteI have same problem and also the text and icons on toolbar doesn't appear .. please some help
DeleteSame problem.. is there any solution?
DeleteThank you so much for this great tutorial. You have explained it so well.
ReplyDeleteHi, thanks for your tutorial!
ReplyDeleteCan you please explain the content of the styles.xml that we have to create for <5.0 version of Android?
This comment has been removed by the author.
ReplyDeleteThumbs up for the good tutorial. I'm trying to port my app to the new material design and followed your steps (until step 9). However I get a NullPointerException on invoking setSupportActionBar(toolbar). Does anyone have the same issue? I would appreciate for a hint. Thanks :).
ReplyDeleteCheck your activity's xml file... you may have missed the include tag..
Deletehi
ReplyDeleteThanks for ur tut its colorPrimaryDark is not working for <5.0 version works only on 5.0 how could i resolve plz help me
Hello,
ReplyDeleteGreat tutorial, but I have one question : when I click on the settings button, the settings box displays on the menu, and not below ? Do you have also this problem ?
Thank you.
Do I miss something?? I follow this great tut but no text (appname) or icons came in my toolbar...
ReplyDeletehttp://gonalroc.com/2015/04/22/why/
Yeah me too,I was wondering if i have miss something here?
DeleteCheck this post for possible solution: https://stackoverflow.com/questions/26813991/actionbar-toolbar-not-showing-on-lollipop-version-of-app/27487096#27487096
DeleteThis comment has been removed by the author.
DeleteThis comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteHi. I've a problem with tollbar that covered by ExpandableListView. I'm using a Relative Layout, the include code and the expandablelistview.
ReplyDeletehey, thanx for dis great tutorial. i just want to konw how to add images instead of text. and change image color based on selection.
ReplyDeleteHi am getting error check this
ReplyDeletejava.lang.RuntimeException: Unable to start activity ComponentInfo{com.example.sudhan.materaildesign/com.example.sudhan.materaildesign.MainActivity}: java.lang.IllegalStateException: You need to use a Theme.AppCompat theme (or descendant) with this activity.
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2114)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2139)
at android.app.ActivityThread.access$700(ActivityThread.java:143)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1241)
at android.os.Handler.dispatchMessage(Handler.java:99)
at android.os.Looper.loop(Looper.java:137)
at android.app.ActivityThread.main(ActivityThread.java:4960)
at java.lang.reflect.Method.invokeNative(Native Method)
at java.lang.reflect.Method.invoke(Method.java:511)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:1038)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:805)
at dalvik.system.NativeStart.main(Native Method)
Caused by: java.lang.IllegalStateException: You need to use a Theme.AppCompat theme (or descendant) with this activity.
at android.support.v7.app.ActionBarActivityDelegate.onCreate(ActionBarActivityDelegate.java:152)
at android.support.v7.app.ActionBarActivityDelegateBase.onCreate(ActionBarActivityDelegateBase.java:149)
at android.support.v7.app.ActionBarActivity.onCreate(ActionBarActivity.java:123)
at com.example.sudhan.materaildesign.MainActivity.onCreate(MainActivity.java:31)
at android.app.Activity.performCreate(Activity.java:5203)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1094)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2078)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2139)
at android.app.ActivityThread.access$700(ActivityThread.java:143)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1241)
at android.os.Handler.dispatchMessage(Handler.java:99)
at android.os.Looper.loop(Looper.java:137)
at android.app.ActivityThread.main(ActivityThread.java:4960)
at java.lang.reflect.Method.invokeNative(Native Method)
at java.lang.reflect.Method.invoke(Method.java:511)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:1038)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:805)
at dalvik.system.NativeStart.main(Native Method)
I have the same error :/
DeleteCan i have the whole project
ReplyDeleteFor the once that want to chance the color in <5.0 you can use toolbar.setTitleTextColor(getResources().getColor(R.color.TextColor));
ReplyDeletein the activity where you want the color.
hi akash,i have followed your great tuts and everything was doing fine until i notice that in the bar have no 'MATERIAL TEXT' in the left side and those '3 dots' in the right side,Am i missing something here..?
ReplyDeleteHere's my mainactivity java looks like:
ReplyDeleteimport android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.support.v7.widget.Toolbar;
import android.view.Menu;
import android.view.MenuItem;
public class MainActivity extends ActionBarActivity {
private Toolbar toolbar;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
toolbar = (Toolbar) findViewById(R.id.tool_bar);
setSupportActionBar(toolbar);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
Hi Akash bangad,
ReplyDeleteI'm getting rendering problems. Can you help me solve this issue.
The following classes could not be instantiated:
- android.support.v7.internal.widget.ActionBarOverlayLayout
(Open Class, Show Exception, Clear Cache)
Tip: Use View.isInEditMode() in your custom views to skip code or show sample data when shown in the IDE Exception Details java.lang.ClassNotFoundException: android.support.v4.view.ViewPropertyAnimatorListener Copy stack to clipboard
This comment has been removed by the author.
ReplyDeleteAfter I change the text color in toolbar ,the setting overflow background become black ,is there any method to keep the background still white? thank you :)
ReplyDeleteThis comment has been removed by the author.
DeleteThis comment has been removed by the author.
DeleteAlright, this time I'm posting without the "smaller/bigger" signs. The code was deleted at my previous comments because of them. I've replaced them with normal brackets: "()". I hope it won't prevent you from understanding the code.
DeleteHere is my tool_bar.xml file:
(?xml version="1.0" encoding="utf-8"?)
(android.support.v7.widget.Toolbar
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/colorPrimary"
app:theme="@style/ThemeOverlay.AppCompat.Dark"
app:popupTheme="@style/ThemeOverlay.AppCompat.Light"
android:elevation="4dp" )
(/android.support.v7.widget.Toolbar)
I've made some changes. I added the "app" attribute (xmlns:app="http://schemas.android.com/apk/res-auto"), and then I used app:theme (instead of android:theme, as described at this article) so that I can use app:popupTheme to color the popup overflow (to make it light). I guess I didn't explain it well, but if you'll look at the code, you'll get the idea.
Exactly, I was looking for.. Thanks mate.. :)
DeleteNice article... can you please post how to have the back button in child activity's toolbar to go to the parent activity. After using the toolbar, the back button is not showing even after adding meta tag in manifest, as it was done before....
ReplyDeleteIt shows a rendering problem.
ReplyDeleteThe following class could not be instantiated-
android.support.v7.widget.Toolbar (Open Class, Show Exception, Clear cache)
Sir
ReplyDeletei have error with this code
**********
private Toolbar toolbar;
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
toolbar = (Toolbar) findViewById(R.id.app_bar);
setSupportActionBar(toolbar); l
}
****************
got an exception android.support.v7 .widget toolbar cannot cast into android.support.toolbar
some fella told me to have backward compatibility u have to import this class file
but i am unable to do this
also i dont want backward compatibilty , i just wanna run on lollipop..
you must have a problem with your imports.
Deleteuse the android.support.v7.widget.toolbar either than android.support.toolbar.
First Thanks for your great tutorial...I learned google studio from 3 days before...Your tutorial was very clear and good to underestand, I did that all but Now I want centering Icon....but couldn;t do that. I asked this question in stackoverflow.com but no one couldn't answer me. please see here: http://stackoverflow.com/questions/31154029/how-to-center-icons-in-toolbar-in-android-studio can you please tell me how centring icons?
ReplyDeletehi akash, nice tutorial!!.. i just ask a question.. do you know why in my toolbar set the name of the package? i want to set with the name of my aplication.. can u give me a clue? thanks again!!
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteGreat tutorials...
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteAwsome for beginer.....
ReplyDeletewhen I use span it shows error that element must be declared how o solve it please help..
ReplyDeletesuperb tutorial
ReplyDeleteHi,
ReplyDeleteI have done the same but when i set the compileSdk to 19 the app crashes.
Please help me figure out what I mught be missing.
Thanks in advance!
Hi i did try this tutorial and its working fine but i just want to know is there any option for changing size of that menu item icon.
ReplyDeleteHi Akash the tutorial was very useful. But the issue i am facing is when i switch the screen orientation the application crashes with following error's
ReplyDelete2389-2389/? E/Parcel﹕ Class not found when unmarshalling: android.support.v7.widget.Toolbar$SavedState
java.lang.ClassNotFoundException: android.support.v7.widget.Toolbar$SavedState...
Caused by: java.lang.NoClassDefFoundError: android/support/v7/widget/Toolbar$Save
Great Tutorial,
ReplyDeleteJust curious why use ActionBarActivity if there is AppCompatAcitivity?
Thanks
ActionBarActivity is now depricated in newer version of Android Studio ..
Deletenow use AppComaptActivity further for the same results
Hello
ReplyDeleteCan we have two action bars? (I mean one at top and one at bottom)
If possible, how?
dear sir
ReplyDeletewhen i run this app then profile image is not showing in app bar
Your tutorials are really helpful.They are written in such a manner that even a non developer can built a good mobile app.I really appreciate.You are a true bloger.
ReplyDeleteThanks
Thanks For Giving your valuable time
ReplyDeleteThanks Great Article. Straight to the point.
ReplyDeleteGreat article. Good job
ReplyDeleteIn activity_main.xml, "Cnnot resolve symbol @dimen/TextDimTop".
ReplyDeleteWhat to do?
This comment has been removed by the author.
ReplyDeletecan we integrate the navigation drawer with actionbar activity in dis project
ReplyDeleteThe toolbar remains blank and doesn't show the title or the action button. I have been looking for a solution but couldn't find one. Any idea anyone?
ReplyDeleteYou are a real hero.
ReplyDeletehow to animate navigation icon on toolbar at 90 degree?
ReplyDeleteHow can set two different theme: one for action bar with (android:theme="@style/ThemeOverlay.AppCompat.Dark") and get a white text title; second for overflow menu to set a AppCompat.Light theme?
ReplyDeleteGuys, what does span tag mean? %)
ReplyDeleteGuys, what does span tag mean? %)
ReplyDeleteThe toolbar remains blank and doesn't show the title or the action button. I have been looking for a solution but couldn't find one. Any idea anyone?
ReplyDeleteAndroid App Development Tutorial: viralandroid.com/2015/11/android-tutorial.html
ReplyDeleteThanks
ReplyDeleteHow to increase size of the item icon?
ReplyDeleteYour webpage programming needs a bit of tweak. There is span in each code section.
ReplyDeletehi sir
ReplyDeletethanks for the valuable notes. i just want some help regarding the same. i have android studio 1.4 and when i select blank activity i get two xml layout files. how can we use the above codes in that respect?
and secondly android studio is not acknowledging Span tag.
Nice explaination :)
ReplyDeleteCan you give me the source code of this project.
ReplyDeleteThanks
No github link?
ReplyDeleteYou have a small bug? Please add
android:layout_below="@+id/tool_bar"
to the TextView attribute section. Without this the tool bar overlaps the TextView content
Nice Article, It clarifies my misunderstanding, simply modify the xml file rather add more lines on java file. it is more convenient to use
ReplyDeletethanks
Awesome tutorial ,you know how icon placed in left side of Toolbar .
ReplyDeleteGreat Article!!!Helps me a lot,but i have a question:-I am using a Navigation Drawer activity and i want to change the color of bars(3 Horizontal lines) that are displayed in the Left Top,How? It will be great if you reply me asap.
ReplyDeleteAt the end of the article: "So finally we have done and our App Bar looks just how we had seen above and as we have used the appcombat support library [...]", appcombat is a typo.
ReplyDeleteVery Nice and Helpful!
ReplyDeletehello sir,
ReplyDeleteI have one question regarding changing status bar.
I find one problem with change status bar color with android studio. but it is working for pragmatically.... I used try {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) {
Window window = getWindow();
window.addFlags(WindowManager.LayoutParams.FLAG_DRAWS_SYSTEM_BAR_BACKGROUNDS);
window.setStatusBarColor(Color.BLUE);
}
} catch (Exception e) {
e.printStackTrace();
}....
it working fine but if i change status bar color with theme then it's not working .. so can you provides any idea where i do wrong...
@color/colorPrimary
@color/colorPrimaryDark
@color/textColorPrimary
looking for response
thanks
Thank You so muuuuuuch , Sir ;
ReplyDeleteI followed your steps , everything works fine except status bar color .status bar color is white (min sdk=21 , emulator uses android 5.0)
Do you know why nothing appears in design mode? The content of Toolbar only apperars in runtime.
ReplyDeleteThanks
hii.. i am in starting phase of android development. And just want to learn Material design can you please give me the link or any material where i can learn about material design.
ReplyDelete
ReplyDeletenight,
thanks for the tutorial. please advice and criticism on my first application
https://drive.google.com/file/d/0B3eiYbiyWsShbEg4SWRxMzdnU2s/view?usp=sharing
thank you
Awesome and Clear tutorial i have ever found! For those who are asking about colorPrimaryDark, well i am just newbie but according to what ive understood, it is matter of framework and system ui built inside of your OS. Pre-lollipop android versions are not designed for that effect where status bar gets darken color than app bar! Correct me if am wrong somewhere!
ReplyDeleteHey fellas Hii
ReplyDeleteI am just at the begineer in Android & i just started Making an Android app & watching your material design videos. I just got stucked in one of the video https://www.youtube.com/watchv=4XfDDfa3rv8&index=4&list=PLonJJ3BVjZW6CtAMbJz1XD8ELUs1KXaTD
https://www.youtube.com/watch?v=EAZv1fP-5TM&index=5&list=PLonJJ3BVjZW6CtAMbJz1XD8ELUs1KXaTD & for that i need help, i'm so much confused. Actually i'm using Android Studio 1.5.1 & all of tutorials mainly works on lesser version. Now you can see that i have 1.5.1, so when i started the material design course without any problem i had made a toolbar/Actionbar but after it i am not getting settings button 3 dot , but in your tutorial it makes automatically & infact when i created & started my application by default our studio give some autogenerated folders & files in that my menu folder is not showing & also there is no file in my project named as menu_main.xml. So please tell me what i will do. Because in any of the tutorial you don't tell us about how you are going to make menu_main.xml file or how you are going to make settings button on toolbar. Kindly help me.
sorry i need to ask
ReplyDeletei cannot extends ActionBarActivity
its default AppCompatActivity
can you tell me why ?
I use Android Studio 1.3.2
and it already have dependencies in gradle file ..
thx anyway ..
i hope you can help me
any way to modify padding or margin of menu items here like it used to be in Actionbar?
ReplyDeletecould u please tell me.. how can implement this in android eclipse?
ReplyDeletehow to impliment search in page (like chrome) for webview
ReplyDeleteHI.
ReplyDeleteAnd for the toolbar has background image Activity? How is the overlay defined?
Thanks for this tutorial. I just stumbled upon this blog and I really liked it. Added to bookmarks
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteI declared it, but the text nor the overflow button show up.
ReplyDeleteHow can I have a Back/Home icon in the Action Bar on left hand side? Could you please provide the details for implementing the same?
ReplyDeleteHow to change the Title of the Action Bar? And can I used it in Fragment? As if each fragment of a single parent activity could have different Action Bar Icons and respective functionality.
ReplyDeletethank you very much for this beautiful tutorial...
ReplyDeleteGreate Tutoiral , really step by step
ReplyDeleteUpdate your tutorials. ActionBarActivity is deprecated. Code gave the error "You need to use a Theme.AppCompat theme (or descendant) with this activity"
ReplyDeleteAwesome tutorial! I'm only beginning to learn how to make Android app but this seemed like a great place to start.
ReplyDeleteI have one question (hopefully you're still active): How would I get the pop-up that appears when I click the Settings icon top be white? Setting the toolbar to the dark theme (to achieve white text) has the effect of giving the popup a grey background.
Nice Article, I have been searching over many sites for the right way to style my toolbar. Thanks for ending my search here !!
ReplyDeleteIdeas and advances, these days are getting obsoletewith every passing moment, henceforth making due in the profession of Java designer resembles strolling on the rope. java
ReplyDeleteWe have assembled various, fruitful stage applications that have development us as one of the world's debut application advancement organizations. Tubemate youtube downloader
ReplyDeleteExcellent!
ReplyDeleteThe numerous enhancements that have occurred incorporate, upgrading equipment speed, camera, camcorder, and photograph exhibition changes, better virtual console, USB tying and WiFi hotspot usefulness.
ReplyDeleteWhatsapp Plus apk
These apps run on the internet. Apps allow the user to any information, play games, learn cooking and so on. There are apps for everything. There are apps on photography, travel, cooking, language, general knowledge, games and so on. So these apps make the smart phone just like a computer. top 10 best android apps to learn programming
ReplyDeleteOw! Awesome post, keep updating this kind of post. Thanks for this post dude.
ReplyDeleteCan you check my blogs also?
Tubemate Video Downloader is one of the best YouTube downloader app.
SnapTube is the best video downloader in 2017.
WifiKill APK is the best WiFi controller app for your data saving way!
Tubemate downloader 2017 is a multi-platform entertainment application with search, download movie, videos or music function from popular web sites such as: Dailymotion, Youtube, Soundcloud ... On these web pages, you can search for the most unique entertainment tools. Tubemate for Android is a utility for you to enjoy watching and downloading videos on your phone.
ReplyDeleteTubemate 2.4.0 support for phones running the Android operating system. The design is compact, so it does not take up much of the device memory. Each video has many different resolutions, the application allows you to choose different resolutions that match your phone's screen from 240p to 1080p, for high-definition phones, you can download and watch Full HD video in high definition. The software also has many formats for you to choose. In particular, it also supports converting video format to Mp3 format.
Key features:
- Upgrade video download feature to phone
- Watch Full HD high speed video
- Interface utility
- Download multiple videos at once
- Convert to Mp3 (powered by Mp3 Media Converter)
- Like and comment videos with your gmail account
- Share videos through Google Buzz, Twitter, email, ...
- Subscribe to your favorite channels
Tubemate for Android is a great solution for you to own favorite videos from Youtube to help you watch offline for free. Videos downloaded on the phone will be viewed at a faster speed than when viewed online. Let the Tubemate for Android clear away your boredom and tiredness.
This was really a very good post. Thanks for the information.
ReplyDeleteBest SEO Training in Bangalore
Fabulous blog. Very well described this informative post by the author. Thanks.
ReplyDeleteFix your technical problem with Fixingblog. We are also providing help for Norton.com/setup.If you have any problem With Router, Range Extender, Antivirus etc.
ReplyDeleteBefore you plan to install antivirus software on your device, you are required to take
few important steps to avoid software conflicts with the previously installed versions. please visit
norton activation code
Try Tamil Movies Download via VidMate APP.
ReplyDeleteYou can also use VidMate for PC to download videos or movies on your PC!
It has been primarily designed for touch-screen mobile devices such as smart phones and tablets and is being increasingly used for other high-end Android applications such as televisions, cars, games consoles, digital cameras, watches, and other electronic equipments.Download ShowBox APK
ReplyDeleteHave you ever tried using a hotmail entrar login account which was introduced during an email service that was based on the Web when it was a novel concept.
ReplyDeleteThank you so much for giving pieces of information. POGO online gaming is a perfect platform to make your dream come true. In case if you
ReplyDeleteforget your Pogo account password or are you not able to recover pogo Account. visit here: Recover Pogo Account Password
This comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteFor good connectivity of our wifi network, we should use the
ReplyDeleteNetgear router for our home and office. To face any problem relate to setup
setup netgear extender with new router
Thank you for your great post of Material Design bar styling.
ReplyDeleteWorking on Android Studio is a very new experience to me.
But i find hectic when i analysis codes of SnapTube app and tutuapp
This post is really helpful for me.
Thanks for sharing this informative post ims
ReplyDeleteHP Printer Offline Support
ReplyDeleteHP Printer Offline Support 1-844-669-3399 USA. Getting Problem with your HP Printer it show offline issue. We are Here to help you any type of troubleshooting HP printer offline problem. Our Technical team 24*7 Ready to fix your HP Printer offline problems.
Get Unlimited Hp printer offline setup Support USA form devices support by just giving a call on +1-844-247-7256. We offer you services according to your issue and fix them as soon as possible.
ReplyDeleteHow to sign up, login and secure your Hotmail - Outlook account. Log on to hotmail.com safely. This article we will guide all.
ReplyDeleteInpormative blog buddy...
ReplyDeletecheck my blog..
Free Download Wifikill Pro Apk
Nice blog.
ReplyDeletemake your backyard safe for kids
very nice
ReplyDelete--------------------
شركة غسيل وتلميع سيارات متنقلة بالرياض
شركة تنظيف سيارات بالبخار أمام المنازل بالرياض
شركة التلميع الساطع للسيارات بالرياض
شركة تنظيف مراتب السيارات بالرياض
شركة عزل حراري وتظليل للسيارات بالرياض
good post
ReplyDelete-------------
شركة مكافحة الارضة بجازان
شركة مكافحة الحشرات بجازان
شركة مكافحة النمل ابيض بنجران
شركات نقل عفش بخميس مشيط
شركة تنظيف منازل بخميس مشيط
شركة نقل عفش بخميس مشيط
Wow, this is fascinating reading. I am glad I found this and got to read it. Great job on this content. I liked it a lot. Thanks for the great and unique info. Text Auto Reply
ReplyDeleteI got too much interesting stuff on your blog. I guess I am not the only one having all the enjoyment here! Keep up the good work. Auto Reply while Driving
ReplyDeleteHey There. I found your blog using msn. This is a very well written article. I’ll be sure to bookmark it and come back to read more of your useful info. Thanks for the post. I’ll definitely return. Auto Reply Text While Driving
ReplyDeleteThats great post !! I like ur every post they always give me some new knowledge.
ReplyDeleteVidMate | VidMate for PC |
VidMate 2014
We would like to thank you for this wonderful article
ReplyDeleteشركة تنظيف خزانات بالخرج
شركة تنظيف مساجد بالخرج
شركة مكافحة حشرات بالخرج
شركة رش مبيدات بالخرج
شركة تسليك مجاري بالخرج
شركة تنظيف ببريدة